mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-30 05:30:51 +00:00
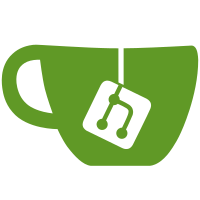
commite712f469a5
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:59:13 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit2a00282308
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:58:30 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit9e06f407c8
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:55:14 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit9581620f1e
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:54:22 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit2a8564c68c
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:47:34 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitcf60632d78
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:40:09 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitee3d1dc6e0
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:22:49 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit65592ef711
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:18:17 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit37972c2573
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:17:06 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitdd909d1499
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:16:28 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit3cf43266ca
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:15:42 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit6731a3df96
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:13:35 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit1b5727e0d5
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:11:18 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitbbcf726a55
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:09:04 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitc03b477d5e
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:06:30 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit2986e2fb04
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:05:44 2017 +0300 Less dependencies [#CLICKHOUSE-2] commit5d6cdef13d
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:04:53 2017 +0300 Less dependencies [#CLICKHOUSE-2] commitf2b819b25c
Author: Alexey Milovidov <milovidov@yandex-team.ru> Date: Sat Jan 14 11:01:47 2017 +0300 Less dependencies [#CLICKHOUSE-2]
44 lines
1.2 KiB
C++
44 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include <string>
|
|
#include <memory>
|
|
#include <unordered_map>
|
|
#include <common/singleton.h>
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
class Context;
|
|
class IFunction;
|
|
using FunctionPtr = std::shared_ptr<IFunction>;
|
|
|
|
|
|
/** Creates function by name.
|
|
* Function could use for initialization (take ownership of shared_ptr, for example)
|
|
* some dictionaries from Context.
|
|
*/
|
|
class FunctionFactory : public Singleton<FunctionFactory>
|
|
{
|
|
friend class StorageSystemFunctions;
|
|
|
|
private:
|
|
typedef FunctionPtr (*Creator)(const Context & context); /// Not std::function, for lower object size and less indirection.
|
|
std::unordered_map<std::string, Creator> functions;
|
|
|
|
public:
|
|
FunctionFactory();
|
|
|
|
FunctionPtr get(const std::string & name, const Context & context) const; /// Throws an exception if not found.
|
|
FunctionPtr tryGet(const std::string & name, const Context & context) const; /// Returns nullptr if not found.
|
|
|
|
/// No locking, you must register all functions before usage of get, tryGet.
|
|
template <typename F> void registerFunction()
|
|
{
|
|
static_assert(std::is_same<decltype(&F::create), Creator>::value, "F::create has incorrect type");
|
|
functions[F::name] = &F::create;
|
|
}
|
|
};
|
|
|
|
}
|