mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-11-05 15:21:43 +00:00
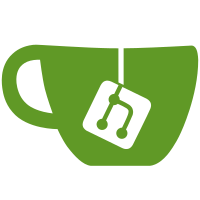
Added: * IDataTypeDomain interface; * method DataTypeFactory::registerDataTypeDomain for registering domains; * DataTypeDomainWithSimpleSerialization domain base class with simple serialization/deserialization; * Concrete IPv4 and IPv6 domain implementations: DataTypeDomanIPv6 and DataTypeDomanIPv4; Updated: * IDataType text serialization/deserialization methods; * IDataType implementation to use domain for text serialization/deserialization; * Refactored implementation of the IPv4/IPv6 functions to use formatIPv4/v6 and parseIPv4/v6 from Common/formatIPv6.h; Tests: * Added test cases for IPv4 and IPv6 domains. * Updated IPv4/v6 functions tests to validate more cases; * Added performance tests for IPv4 and IPv6 related functions;
68 lines
1.6 KiB
C++
68 lines
1.6 KiB
C++
#include <IO/WriteHelpers.h>
|
|
#include <IO/WriteBufferValidUTF8.h>
|
|
#include <Formats/JSONEachRowRowOutputStream.h>
|
|
#include <Formats/FormatFactory.h>
|
|
#include <Formats/BlockOutputStreamFromRowOutputStream.h>
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
|
|
JSONEachRowRowOutputStream::JSONEachRowRowOutputStream(WriteBuffer & ostr_, const Block & sample, const FormatSettings & settings)
|
|
: ostr(ostr_), settings(settings)
|
|
{
|
|
size_t columns = sample.columns();
|
|
fields.resize(columns);
|
|
|
|
for (size_t i = 0; i < columns; ++i)
|
|
{
|
|
WriteBufferFromString out(fields[i]);
|
|
writeJSONString(sample.getByPosition(i).name, out, settings);
|
|
}
|
|
}
|
|
|
|
|
|
void JSONEachRowRowOutputStream::writeField(const IColumn & column, const IDataType & type, size_t row_num)
|
|
{
|
|
writeString(fields[field_number], ostr);
|
|
writeChar(':', ostr);
|
|
type.serializeAsTextJSON(column, row_num, ostr, settings);
|
|
++field_number;
|
|
}
|
|
|
|
|
|
void JSONEachRowRowOutputStream::writeFieldDelimiter()
|
|
{
|
|
writeChar(',', ostr);
|
|
}
|
|
|
|
|
|
void JSONEachRowRowOutputStream::writeRowStartDelimiter()
|
|
{
|
|
writeChar('{', ostr);
|
|
}
|
|
|
|
|
|
void JSONEachRowRowOutputStream::writeRowEndDelimiter()
|
|
{
|
|
writeCString("}\n", ostr);
|
|
field_number = 0;
|
|
}
|
|
|
|
|
|
void registerOutputFormatJSONEachRow(FormatFactory & factory)
|
|
{
|
|
factory.registerOutputFormat("JSONEachRow", [](
|
|
WriteBuffer & buf,
|
|
const Block & sample,
|
|
const Context &,
|
|
const FormatSettings & format_settings)
|
|
{
|
|
return std::make_shared<BlockOutputStreamFromRowOutputStream>(
|
|
std::make_shared<JSONEachRowRowOutputStream>(buf, sample, format_settings), sample);
|
|
});
|
|
}
|
|
|
|
}
|