mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-20 16:50:48 +00:00
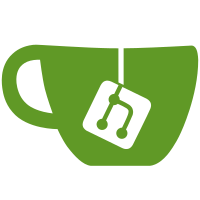
This PR formats all the `*.py` files found under the `tests/integration` folder. It also reorders the imports and cleans up a bunch of unused imports. The formatting also takes care of other things like wrapping lines and fixing spaces and indents such that the tests look more readable.
38 lines
1.3 KiB
Python
38 lines
1.3 KiB
Python
import pytest
|
|
from helpers.cluster import ClickHouseCluster
|
|
|
|
cluster = ClickHouseCluster(__file__)
|
|
instance = cluster.add_instance('instance')
|
|
|
|
|
|
@pytest.fixture(scope="module", autouse=True)
|
|
def setup_nodes():
|
|
try:
|
|
cluster.start()
|
|
|
|
instance.query("CREATE USER sasha")
|
|
instance.query("CREATE USER masha IDENTIFIED BY 'qwerty'")
|
|
|
|
yield cluster
|
|
|
|
finally:
|
|
cluster.shutdown()
|
|
|
|
|
|
def test_authentication_pass():
|
|
assert instance.query("SELECT currentUser()", user='sasha') == 'sasha\n'
|
|
assert instance.query("SELECT currentUser()", user='masha', password='qwerty') == 'masha\n'
|
|
|
|
# 'no_password' authentication type allows to login with any password.
|
|
assert instance.query("SELECT currentUser()", user='sasha', password='something') == 'sasha\n'
|
|
assert instance.query("SELECT currentUser()", user='sasha', password='something2') == 'sasha\n'
|
|
|
|
|
|
def test_authentication_fail():
|
|
# User doesn't exist.
|
|
assert "vasya: Authentication failed" in instance.query_and_get_error("SELECT currentUser()", user='vasya')
|
|
|
|
# Wrong password.
|
|
assert "masha: Authentication failed" in instance.query_and_get_error("SELECT currentUser()", user='masha',
|
|
password='123')
|