mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-11-18 21:51:57 +00:00
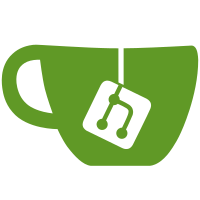
This PR formats all the `*.py` files found under the `tests/integration` folder. It also reorders the imports and cleans up a bunch of unused imports. The formatting also takes care of other things like wrapping lines and fixing spaces and indents such that the tests look more readable.
87 lines
3.4 KiB
Python
87 lines
3.4 KiB
Python
import pytest
|
|
|
|
from helpers.cluster import ClickHouseCluster
|
|
|
|
cluster = ClickHouseCluster(__file__)
|
|
node = cluster.add_instance('node', main_configs=['configs/remote_servers.xml'])
|
|
|
|
cluster_param = pytest.mark.parametrize("cluster", [
|
|
('test_cluster'),
|
|
('test_cluster_2'),
|
|
])
|
|
|
|
|
|
@pytest.fixture(scope="module")
|
|
def started_cluster():
|
|
try:
|
|
cluster.start()
|
|
node.query("create database test engine=Ordinary")
|
|
yield cluster
|
|
|
|
finally:
|
|
cluster.shutdown()
|
|
|
|
|
|
@cluster_param
|
|
def test_single_file(started_cluster, cluster):
|
|
node.query(
|
|
"create table test.distr_1 (x UInt64, s String) engine = Distributed('{}', database, table)".format(cluster))
|
|
node.query("insert into test.distr_1 values (1, 'a'), (2, 'bb'), (3, 'ccc')",
|
|
settings={"use_compact_format_in_distributed_parts_names": "1"})
|
|
|
|
query = "select * from file('/var/lib/clickhouse/data/test/distr_1/shard1_replica1/1.bin', 'Distributed')"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
query = "create table t (dummy UInt32) engine = File('Distributed', '/var/lib/clickhouse/data/test/distr_1/shard1_replica1/1.bin');" \
|
|
"select * from t"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
node.query("drop table test.distr_1")
|
|
|
|
|
|
@cluster_param
|
|
def test_two_files(started_cluster, cluster):
|
|
node.query(
|
|
"create table test.distr_2 (x UInt64, s String) engine = Distributed('{}', database, table)".format(cluster))
|
|
node.query("insert into test.distr_2 values (0, '_'), (1, 'a')",
|
|
settings={"use_compact_format_in_distributed_parts_names": "1"})
|
|
node.query("insert into test.distr_2 values (2, 'bb'), (3, 'ccc')",
|
|
settings={"use_compact_format_in_distributed_parts_names": "1"})
|
|
|
|
query = "select * from file('/var/lib/clickhouse/data/test/distr_2/shard1_replica1/{1,2,3,4}.bin', 'Distributed') order by x"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '0\t_\n1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
query = "create table t (dummy UInt32) engine = File('Distributed', '/var/lib/clickhouse/data/test/distr_2/shard1_replica1/{1,2,3,4}.bin');" \
|
|
"select * from t order by x"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '0\t_\n1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
node.query("drop table test.distr_2")
|
|
|
|
|
|
@cluster_param
|
|
def test_single_file_old(started_cluster, cluster):
|
|
node.query(
|
|
"create table test.distr_3 (x UInt64, s String) engine = Distributed('{}', database, table)".format(cluster))
|
|
node.query("insert into test.distr_3 values (1, 'a'), (2, 'bb'), (3, 'ccc')")
|
|
|
|
query = "select * from file('/var/lib/clickhouse/data/test/distr_3/default@not_existing:9000/1.bin', 'Distributed')"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
query = "create table t (dummy UInt32) engine = File('Distributed', '/var/lib/clickhouse/data/test/distr_3/default@not_existing:9000/1.bin');" \
|
|
"select * from t"
|
|
out = node.exec_in_container(['/usr/bin/clickhouse', 'local', '--stacktrace', '-q', query])
|
|
|
|
assert out == '1\ta\n2\tbb\n3\tccc\n'
|
|
|
|
node.query("drop table test.distr_3")
|