mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-30 13:40:50 +00:00
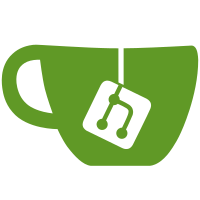
* Add ATTACH PARTITION FROM table for MergeTree. [#CLICKHOUSE-3546] * Implemented replicated case on non-leader replica. [#CLICKHOUSE-3546] * Disable merges in the dropping range. [#CLICKHOUSE-3546] * DROP PARTITION is atomic and simpler now. [#CLICKHOUSE-3546] * Implemented more SYSTEM queries. [#CLICKHOUSE-2931] [#CLICKHOUSE-3546] SYSTEM queries: RESTART REPLICAS SYNC REPLICA db.name STOP MERGES [db.name] START MERGES [db.name] STOP FETCHES [db.name] START FETCHES [db.name] STOP REPLICATED SENDS [db.name] START REPLICATED SENDS [db.name] STOP REPLICATION QUEUES [db.name] START REPLICATION QUEUES [db.name] * Fixed a bunch of bugs in REPLACE PARTITION. [#CLICKHOUSE-3546] * Add tests for REPLACE PARTITION and SYSTEM. [#CLICKHOUSE-3546] * Add system.part_log logging. [#CLICKHOUSE-3546] * Fixed long wait in SYNC REPLICA. [#CLICKHOUSE-3546] * Add requested changes. [#CLICKHOUSE-3546] Fixed clickhouse-client bad return code. * Add requested chenges. [#CLICKHOUSE-3546] * Add requested chenges. [#CLICKHOUSE-3546]
98 lines
2.7 KiB
C++
98 lines
2.7 KiB
C++
#include "ActionLocksManager.h"
|
|
#include <Interpreters/Context.h>
|
|
#include <Databases/IDatabase.h>
|
|
#include <Storages/IStorage.h>
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
namespace ActionLocks
|
|
{
|
|
extern const StorageActionBlockType PartsMerge = 1;
|
|
extern const StorageActionBlockType PartsFetch = 2;
|
|
extern const StorageActionBlockType PartsSend = 3;
|
|
extern const StorageActionBlockType ReplicationQueue = 4;
|
|
}
|
|
|
|
|
|
template <typename F>
|
|
inline void forEachTable(Context & context, F && f)
|
|
{
|
|
for (auto & elem : context.getDatabases())
|
|
for (auto iterator = elem.second->getIterator(context); iterator->isValid(); iterator->next())
|
|
f(iterator->table());
|
|
|
|
}
|
|
|
|
void ActionLocksManager::add(StorageActionBlockType action_type)
|
|
{
|
|
forEachTable(global_context, [&] (const StoragePtr & table)
|
|
{
|
|
ActionLock action_lock = table->getActionLock(action_type);
|
|
|
|
if (!action_lock.expired())
|
|
{
|
|
std::lock_guard<std::mutex> lock(mutex);
|
|
storage_locks[table.get()][action_type] = std::move(action_lock);
|
|
}
|
|
});
|
|
}
|
|
|
|
void ActionLocksManager::add(const String & database_name, const String & table_name, StorageActionBlockType action_type)
|
|
{
|
|
if (auto table = global_context.tryGetTable(database_name, table_name))
|
|
{
|
|
ActionLock action_lock = table->getActionLock(action_type);
|
|
|
|
if (!action_lock.expired())
|
|
{
|
|
std::lock_guard<std::mutex> lock(mutex);
|
|
storage_locks[table.get()][action_type] = std::move(action_lock);
|
|
}
|
|
}
|
|
}
|
|
|
|
void ActionLocksManager::remove(StorageActionBlockType action_type)
|
|
{
|
|
std::lock_guard<std::mutex> lock(mutex);
|
|
|
|
for (auto & storage_elem : storage_locks)
|
|
storage_elem.second.erase(action_type);
|
|
}
|
|
|
|
void ActionLocksManager::remove(const String & database_name, const String & table_name, StorageActionBlockType action_type)
|
|
{
|
|
if (auto table = global_context.tryGetTable(database_name, table_name))
|
|
{
|
|
std::lock_guard<std::mutex> lock(mutex);
|
|
|
|
if (storage_locks.count(table.get()))
|
|
storage_locks[table.get()].erase(action_type);
|
|
}
|
|
}
|
|
|
|
void ActionLocksManager::cleanExpired()
|
|
{
|
|
std::lock_guard<std::mutex> lock(mutex);
|
|
|
|
for (auto it_storage = storage_locks.begin(); it_storage != storage_locks.end(); )
|
|
{
|
|
auto & locks = it_storage->second;
|
|
for (auto it_lock = locks.begin(); it_lock != locks.end(); )
|
|
{
|
|
if (it_lock->second.expired())
|
|
it_lock = locks.erase(it_lock);
|
|
else
|
|
++it_lock;
|
|
}
|
|
|
|
if (locks.empty())
|
|
it_storage = storage_locks.erase(it_storage);
|
|
else
|
|
++it_storage;
|
|
}
|
|
}
|
|
|
|
}
|