mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-11-17 21:24:28 +00:00
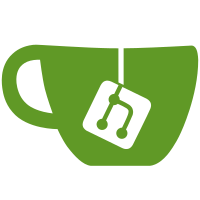
The following headers are pretty generic, so use forward declaration as much as possible: - Context.h - Settings.h - ConnectionTimeouts.h (Also this shows that some missing some includes -- this has been fixed) And split ConnectionTimeouts.h into ConnectionTimeoutsContext.h (since module part cannot be added for it, due to recursive build dependencies that will be introduced) Also remove Settings from the RemoteBlockInputStream/RemoteQueryExecutor and just pass the context, since settings was passed only in speicifc places, that can allow making a copy of Context (i.e. Copier). Approx results (How much units will be recompiled after changing file X?): - ConnectionTimeouts.h - mainline: 100 - Context.h: - mainline: ~800 - patched: 415 - Settings.h: - mainline: 900-1K - patched: 440 (most of them because of the Context.h)
153 lines
4.9 KiB
C++
153 lines
4.9 KiB
C++
#pragma once
|
|
|
|
#include <common/types.h>
|
|
#include <Columns/IColumn.h>
|
|
#include <DataStreams/IBlockStream_fwd.h>
|
|
#include <Formats/FormatSettings.h>
|
|
#include <IO/BufferWithOwnMemory.h>
|
|
|
|
#include <functional>
|
|
#include <memory>
|
|
#include <unordered_map>
|
|
#include <boost/noncopyable.hpp>
|
|
|
|
namespace DB
|
|
{
|
|
|
|
class Block;
|
|
class Context;
|
|
struct Settings;
|
|
struct FormatFactorySettings;
|
|
|
|
class ReadBuffer;
|
|
class WriteBuffer;
|
|
|
|
class IProcessor;
|
|
using ProcessorPtr = std::shared_ptr<IProcessor>;
|
|
|
|
class IInputFormat;
|
|
class IOutputFormat;
|
|
|
|
struct RowInputFormatParams;
|
|
struct RowOutputFormatParams;
|
|
|
|
using InputFormatPtr = std::shared_ptr<IInputFormat>;
|
|
using OutputFormatPtr = std::shared_ptr<IOutputFormat>;
|
|
|
|
FormatSettings getFormatSettings(const Context & context);
|
|
|
|
template <typename T>
|
|
FormatSettings getFormatSettings(const Context & context,
|
|
const T & settings);
|
|
|
|
/** Allows to create an IBlockInputStream or IBlockOutputStream by the name of the format.
|
|
* Note: format and compression are independent things.
|
|
*/
|
|
class FormatFactory final : private boost::noncopyable
|
|
{
|
|
public:
|
|
/// This callback allows to perform some additional actions after reading a single row.
|
|
/// It's initial purpose was to extract payload for virtual columns from Kafka Consumer ReadBuffer.
|
|
using ReadCallback = std::function<void()>;
|
|
|
|
/** Fast reading data from buffer and save result to memory.
|
|
* Reads at least min_chunk_bytes and some more until the end of the chunk, depends on the format.
|
|
* Used in ParallelParsingBlockInputStream.
|
|
*/
|
|
using FileSegmentationEngine = std::function<bool(
|
|
ReadBuffer & buf,
|
|
DB::Memory<> & memory,
|
|
size_t min_chunk_bytes)>;
|
|
|
|
/// This callback allows to perform some additional actions after writing a single row.
|
|
/// It's initial purpose was to flush Kafka message for each row.
|
|
using WriteCallback = std::function<void(
|
|
const Columns & columns,
|
|
size_t row)>;
|
|
|
|
private:
|
|
using InputCreator = std::function<BlockInputStreamPtr(
|
|
ReadBuffer & buf,
|
|
const Block & sample,
|
|
UInt64 max_block_size,
|
|
ReadCallback callback,
|
|
const FormatSettings & settings)>;
|
|
|
|
using OutputCreator = std::function<BlockOutputStreamPtr(
|
|
WriteBuffer & buf,
|
|
const Block & sample,
|
|
WriteCallback callback,
|
|
const FormatSettings & settings)>;
|
|
|
|
using InputProcessorCreator = std::function<InputFormatPtr(
|
|
ReadBuffer & buf,
|
|
const Block & header,
|
|
const RowInputFormatParams & params,
|
|
const FormatSettings & settings)>;
|
|
|
|
using OutputProcessorCreator = std::function<OutputFormatPtr(
|
|
WriteBuffer & buf,
|
|
const Block & sample,
|
|
const RowOutputFormatParams & params,
|
|
const FormatSettings & settings)>;
|
|
|
|
struct Creators
|
|
{
|
|
InputCreator input_creator;
|
|
OutputCreator output_creator;
|
|
InputProcessorCreator input_processor_creator;
|
|
OutputProcessorCreator output_processor_creator;
|
|
FileSegmentationEngine file_segmentation_engine;
|
|
};
|
|
|
|
using FormatsDictionary = std::unordered_map<String, Creators>;
|
|
|
|
public:
|
|
static FormatFactory & instance();
|
|
|
|
BlockInputStreamPtr getInput(
|
|
const String & name,
|
|
ReadBuffer & buf,
|
|
const Block & sample,
|
|
const Context & context,
|
|
UInt64 max_block_size,
|
|
const std::optional<FormatSettings> & format_settings = std::nullopt) const;
|
|
|
|
BlockOutputStreamPtr getOutput(const String & name, WriteBuffer & buf,
|
|
const Block & sample, const Context & context, WriteCallback callback = {},
|
|
const std::optional<FormatSettings> & format_settings = std::nullopt) const;
|
|
|
|
InputFormatPtr getInputFormat(
|
|
const String & name,
|
|
ReadBuffer & buf,
|
|
const Block & sample,
|
|
const Context & context,
|
|
UInt64 max_block_size,
|
|
const std::optional<FormatSettings> & format_settings = std::nullopt) const;
|
|
|
|
OutputFormatPtr getOutputFormat(
|
|
const String & name, WriteBuffer & buf, const Block & sample,
|
|
const Context & context, WriteCallback callback = {},
|
|
const std::optional<FormatSettings> & format_settings = std::nullopt) const;
|
|
|
|
/// Register format by its name.
|
|
void registerInputFormat(const String & name, InputCreator input_creator);
|
|
void registerOutputFormat(const String & name, OutputCreator output_creator);
|
|
void registerFileSegmentationEngine(const String & name, FileSegmentationEngine file_segmentation_engine);
|
|
|
|
void registerInputFormatProcessor(const String & name, InputProcessorCreator input_creator);
|
|
void registerOutputFormatProcessor(const String & name, OutputProcessorCreator output_creator);
|
|
|
|
const FormatsDictionary & getAllFormats() const
|
|
{
|
|
return dict;
|
|
}
|
|
|
|
private:
|
|
FormatsDictionary dict;
|
|
|
|
const Creators & getCreators(const String & name) const;
|
|
};
|
|
|
|
}
|