mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-11-06 07:32:27 +00:00
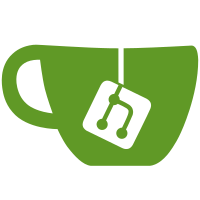
* Remove config_common.h * Refactor libcpuid contrib * Remove support for libcpuinfo * Define USE_CPUID in Arcadia * Refactor Poco libraries
58 lines
953 B
C++
58 lines
953 B
C++
#pragma once
|
|
|
|
#include <new>
|
|
#include "defines.h"
|
|
|
|
#if USE_JEMALLOC
|
|
# include <jemalloc/jemalloc.h>
|
|
#endif
|
|
|
|
#if !USE_JEMALLOC || JEMALLOC_VERSION_MAJOR < 4
|
|
# include <cstdlib>
|
|
#endif
|
|
|
|
|
|
namespace Memory
|
|
{
|
|
|
|
inline ALWAYS_INLINE void * newImpl(std::size_t size)
|
|
{
|
|
auto * ptr = malloc(size);
|
|
if (likely(ptr != nullptr))
|
|
return ptr;
|
|
|
|
/// @note no std::get_new_handler logic implemented
|
|
throw std::bad_alloc{};
|
|
}
|
|
|
|
inline ALWAYS_INLINE void * newNoExept(std::size_t size) noexcept
|
|
{
|
|
return malloc(size);
|
|
}
|
|
|
|
inline ALWAYS_INLINE void deleteImpl(void * ptr) noexcept
|
|
{
|
|
free(ptr);
|
|
}
|
|
|
|
#if USE_JEMALLOC && JEMALLOC_VERSION_MAJOR >= 4
|
|
|
|
inline ALWAYS_INLINE void deleteSized(void * ptr, std::size_t size) noexcept
|
|
{
|
|
if (unlikely(ptr == nullptr))
|
|
return;
|
|
|
|
sdallocx(ptr, size, 0);
|
|
}
|
|
|
|
#else
|
|
|
|
inline ALWAYS_INLINE void deleteSized(void * ptr, std::size_t size [[maybe_unused]]) noexcept
|
|
{
|
|
free(ptr);
|
|
}
|
|
|
|
#endif
|
|
|
|
}
|