mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-20 08:40:50 +00:00
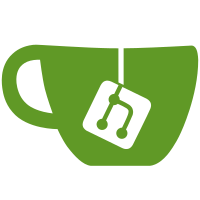
- Mark getCreateTableQuery() non virtual to call it from ctor - Make check for CREATE TABLE simpler (by caching result) - Extend error message. v2: at SystemLog tables has not been initialized yet, so SHOW CREATE cannot be obtained for the existing table, move the check to the prepareTable() call. v3: reset UUID and SETTINGS of MergeTree in CREATE TABLE query v4: fix re-creating table with the same UUID
71 lines
2.5 KiB
Python
71 lines
2.5 KiB
Python
# pylint: disable=line-too-long
|
|
# pylint: disable=unused-argument
|
|
# pylint: disable=redefined-outer-name
|
|
|
|
import pytest
|
|
from helpers.cluster import ClickHouseCluster
|
|
|
|
cluster = ClickHouseCluster(__file__)
|
|
node = cluster.add_instance('node_default', stay_alive=True)
|
|
|
|
@pytest.fixture(scope='module', autouse=True)
|
|
def start_cluster():
|
|
try:
|
|
cluster.start()
|
|
yield cluster
|
|
finally:
|
|
cluster.shutdown()
|
|
|
|
|
|
def test_system_logs_recreate():
|
|
system_logs = [
|
|
# enabled by default
|
|
'query_log',
|
|
'query_thread_log',
|
|
'part_log',
|
|
'trace_log',
|
|
'metric_log',
|
|
]
|
|
|
|
node.query('SYSTEM FLUSH LOGS')
|
|
for table in system_logs:
|
|
assert 'ENGINE = MergeTree' in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert 'ENGINE = Null' not in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert len(node.query(f"SHOW TABLES FROM system LIKE '{table}%'").strip().split('\n')) == 1
|
|
|
|
# NOTE: we use zzz- prefix to make it the last file,
|
|
# so that it will be applied last.
|
|
for table in system_logs:
|
|
node.exec_in_container(['bash', '-c', f"""echo "
|
|
<clickhouse>
|
|
<{table}>
|
|
<engine>ENGINE = Null</engine>
|
|
<partition_by remove='remove'/>
|
|
</{table}>
|
|
</clickhouse>
|
|
" > /etc/clickhouse-server/config.d/zzz-override-{table}.xml
|
|
"""])
|
|
|
|
node.restart_clickhouse()
|
|
node.query('SYSTEM FLUSH LOGS')
|
|
for table in system_logs:
|
|
assert 'ENGINE = MergeTree' not in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert 'ENGINE = Null' in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert len(node.query(f"SHOW TABLES FROM system LIKE '{table}%'").strip().split('\n')) == 2
|
|
|
|
for table in system_logs:
|
|
node.exec_in_container(['rm', f'/etc/clickhouse-server/config.d/zzz-override-{table}.xml'])
|
|
|
|
node.restart_clickhouse()
|
|
node.query('SYSTEM FLUSH LOGS')
|
|
for table in system_logs:
|
|
assert 'ENGINE = MergeTree' in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert 'ENGINE = Null' not in node.query(f'SHOW CREATE TABLE system.{table}')
|
|
assert len(node.query(f"SHOW TABLES FROM system LIKE '{table}%'").strip().split('\n')) == 3
|
|
|
|
node.query('SYSTEM FLUSH LOGS')
|
|
# Ensure that there was no superfluous RENAME's
|
|
# IOW that the table created only when the structure is indeed different.
|
|
for table in system_logs:
|
|
assert len(node.query(f"SHOW TABLES FROM system LIKE '{table}%'").strip().split('\n')) == 3
|