mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-30 05:30:51 +00:00
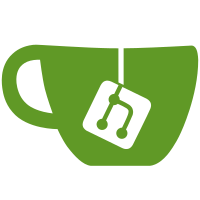
It's still hackish and dirty, but server and client compies. Server starts, but throwes meaningless exception on any query. Client seems to be working fine. Linux compilation might (but shouldn't) be broken (not tested).
70 lines
2.2 KiB
C++
70 lines
2.2 KiB
C++
#pragma once
|
|
|
|
#include <DB/Interpreters/Context.h>
|
|
#include <DB/Dictionaries/DictionaryStructure.h>
|
|
#include <DB/Dictionaries/IDictionarySource.h>
|
|
#include <DB/Dictionaries/OwningBufferBlockInputStream.h>
|
|
#include <DB/IO/ReadBufferFromFile.h>
|
|
#include <Poco/Timestamp.h>
|
|
#include <Poco/File.h>
|
|
|
|
namespace DB
|
|
{
|
|
|
|
/// Allows loading dictionaries from a file with given format, does not support "random access"
|
|
class FileDictionarySource final : public IDictionarySource
|
|
{
|
|
static const auto max_block_size = 8192;
|
|
|
|
public:
|
|
FileDictionarySource(const std::string & filename, const std::string & format, Block & sample_block,
|
|
const Context & context)
|
|
: filename{filename}, format{format}, sample_block{sample_block}, context(context)
|
|
{}
|
|
|
|
FileDictionarySource(const FileDictionarySource & other)
|
|
: filename{other.filename}, format{other.format},
|
|
sample_block{other.sample_block}, context(other.context),
|
|
last_modification{other.last_modification}
|
|
{}
|
|
|
|
BlockInputStreamPtr loadAll() override
|
|
{
|
|
auto in_ptr = std::make_unique<ReadBufferFromFile>(filename);
|
|
auto stream = context.getInputFormat(
|
|
format, *in_ptr, sample_block, max_block_size);
|
|
last_modification = getLastModification();
|
|
|
|
return std::make_shared<OwningBufferBlockInputStream>(stream, std::move(in_ptr));
|
|
}
|
|
|
|
BlockInputStreamPtr loadIds(const std::vector<UInt64> & ids) override
|
|
{
|
|
throw Exception{"Method unsupported", ErrorCodes::NOT_IMPLEMENTED};
|
|
}
|
|
|
|
BlockInputStreamPtr loadKeys(
|
|
const ConstColumnPlainPtrs & key_columns, const std::vector<std::size_t> & requested_rows) override
|
|
{
|
|
throw Exception{"Method unsupported", ErrorCodes::NOT_IMPLEMENTED};
|
|
}
|
|
|
|
bool isModified() const override { return getLastModification() > last_modification; }
|
|
bool supportsSelectiveLoad() const override { return false; }
|
|
|
|
DictionarySourcePtr clone() const override { return std::make_unique<FileDictionarySource>(*this); }
|
|
|
|
std::string toString() const override { return "File: " + filename + ' ' + format; }
|
|
|
|
private:
|
|
Poco::Timestamp getLastModification() const { return Poco::File{filename}.getLastModified(); }
|
|
|
|
const std::string filename;
|
|
const std::string format;
|
|
Block sample_block;
|
|
const Context & context;
|
|
Poco::Timestamp last_modification;
|
|
};
|
|
|
|
}
|