mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-30 05:30:51 +00:00
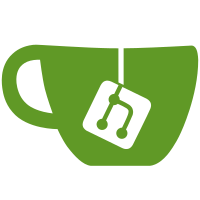
* Add ATTACH PARTITION FROM table for MergeTree. [#CLICKHOUSE-3546] * Implemented replicated case on non-leader replica. [#CLICKHOUSE-3546] * Disable merges in the dropping range. [#CLICKHOUSE-3546] * DROP PARTITION is atomic and simpler now. [#CLICKHOUSE-3546] * Implemented more SYSTEM queries. [#CLICKHOUSE-2931] [#CLICKHOUSE-3546] SYSTEM queries: RESTART REPLICAS SYNC REPLICA db.name STOP MERGES [db.name] START MERGES [db.name] STOP FETCHES [db.name] START FETCHES [db.name] STOP REPLICATED SENDS [db.name] START REPLICATED SENDS [db.name] STOP REPLICATION QUEUES [db.name] START REPLICATION QUEUES [db.name] * Fixed a bunch of bugs in REPLACE PARTITION. [#CLICKHOUSE-3546] * Add tests for REPLACE PARTITION and SYSTEM. [#CLICKHOUSE-3546] * Add system.part_log logging. [#CLICKHOUSE-3546] * Fixed long wait in SYNC REPLICA. [#CLICKHOUSE-3546] * Add requested changes. [#CLICKHOUSE-3546] Fixed clickhouse-client bad return code. * Add requested chenges. [#CLICKHOUSE-3546] * Add requested chenges. [#CLICKHOUSE-3546]
46 lines
1.2 KiB
C++
46 lines
1.2 KiB
C++
#pragma once
|
|
#include <Core/Types.h>
|
|
#include <Common/ActionLock.h>
|
|
#include <unordered_map>
|
|
#include <mutex>
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
class IStorage;
|
|
class Context;
|
|
|
|
/// Holds ActionLocks for tables
|
|
/// Does not store pointers to tables
|
|
class ActionLocksManager
|
|
{
|
|
public:
|
|
explicit ActionLocksManager(Context & global_context_) : global_context(global_context_) {}
|
|
|
|
/// Adds new locks for each table
|
|
void add(StorageActionBlockType action_type);
|
|
/// Add new lock for a table if it has not been already added
|
|
void add(const String & database_name, const String & table_name, StorageActionBlockType action_type);
|
|
|
|
/// Remove locks for all tables
|
|
void remove(StorageActionBlockType action_type);
|
|
/// Removes a lock for a table if it exists
|
|
void remove(const String & database_name, const String & table_name, StorageActionBlockType action_type);
|
|
|
|
/// Removes all locks of non-existing tables
|
|
void cleanExpired();
|
|
|
|
private:
|
|
Context & global_context;
|
|
|
|
using StorageRawPtr = const IStorage *;
|
|
using Locks = std::unordered_map<size_t, ActionLock>;
|
|
using StorageLocks = std::unordered_map<StorageRawPtr, Locks>;
|
|
|
|
mutable std::mutex mutex;
|
|
StorageLocks storage_locks;
|
|
};
|
|
|
|
}
|