mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-12-15 02:41:59 +00:00
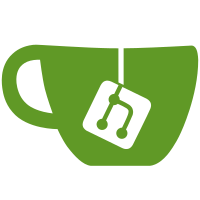
Public suffix list may contain special characters (you may find format here - [1]): - asterisk (*) - exclamation mark (!) [1]: https://github.com/publicsuffix/list/wiki/Format It is easier to describe how it should be interpreted with an examples. Consider the following part of the list: *.sch.uk *.kawasaki.jp !city.kawasaki.jp And here are the results for `cutToFirstSignificantSubdomainCustom()`: If you have only asterisk (*): foo.something.sheffield.sch.uk -> something.sheffield.sch.uk sheffield.sch.uk -> sheffield.sch.uk If you have exclamation mark (!) too: foo.kawasaki.jp -> foo.kawasaki.jp foo.foo.kawasaki.jp -> foo.foo.kawasaki.jp city.kawasaki.jp -> city.kawasaki.jp some.city.kawasaki.jp -> city.kawasaki.jp TLDs had been verified wit the following script [2], to match with python publicsuffix2 module. [2]: https://gist.github.com/azat/c1a7a9f1e3519793134ef4b1df5461a6 v2: fix StringHashTable padding requirements Fixes: #39468 Follow-up for: #17748 Signed-off-by: Azat Khuzhin <a.khuzhin@semrush.com>
81 lines
1.9 KiB
C++
81 lines
1.9 KiB
C++
#pragma once
|
|
|
|
#include <base/defines.h>
|
|
#include <base/StringRef.h>
|
|
#include <Common/HashTable/StringHashMap.h>
|
|
#include <Common/Arena.h>
|
|
#include <Poco/Util/AbstractConfiguration.h>
|
|
#include <mutex>
|
|
#include <string>
|
|
#include <unordered_map>
|
|
|
|
namespace DB
|
|
{
|
|
|
|
enum TLDType
|
|
{
|
|
/// Does not exist marker
|
|
TLD_NONE,
|
|
/// For regular lines
|
|
TLD_REGULAR,
|
|
/// For asterisk (*)
|
|
TLD_ANY,
|
|
/// For exclamation mark (!)
|
|
TLD_EXCLUDE,
|
|
};
|
|
|
|
/// Custom TLD List
|
|
///
|
|
/// Unlike tldLookup (which uses gperf) this one uses plain StringHashMap.
|
|
class TLDList
|
|
{
|
|
public:
|
|
using Container = StringHashMap<TLDType>;
|
|
|
|
explicit TLDList(size_t size);
|
|
|
|
void insert(const String & host, TLDType type);
|
|
TLDType lookup(StringRef host) const;
|
|
size_t size() const { return tld_container.size(); }
|
|
|
|
private:
|
|
Container tld_container;
|
|
std::unique_ptr<Arena> memory_pool;
|
|
};
|
|
|
|
class TLDListsHolder
|
|
{
|
|
public:
|
|
using Map = std::unordered_map<std::string, TLDList>;
|
|
|
|
static TLDListsHolder & getInstance();
|
|
|
|
/// Parse "top_level_domains_lists" section,
|
|
/// And add each found dictionary.
|
|
void parseConfig(const std::string & top_level_domains_path, const Poco::Util::AbstractConfiguration & config);
|
|
|
|
/// Parse file and add it as a Set to the list of TLDs
|
|
/// - "//" -- comment,
|
|
/// - empty lines will be ignored.
|
|
///
|
|
/// Treats the following special symbols:
|
|
/// - "*"
|
|
/// - "!"
|
|
///
|
|
/// Format : https://github.com/publicsuffix/list/wiki/Format
|
|
/// Example: https://publicsuffix.org/list/public_suffix_list.dat
|
|
///
|
|
/// Return size of the list.
|
|
size_t parseAndAddTldList(const std::string & name, const std::string & path);
|
|
/// Throws TLD_LIST_NOT_FOUND if list does not exist
|
|
const TLDList & getTldList(const std::string & name);
|
|
|
|
protected:
|
|
TLDListsHolder();
|
|
|
|
std::mutex tld_lists_map_mutex;
|
|
Map tld_lists_map TSA_GUARDED_BY(tld_lists_map_mutex);
|
|
};
|
|
|
|
}
|