mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-20 08:40:50 +00:00
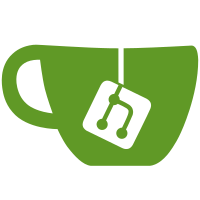
- TSA is a static analyzer build by Google which finds race conditions and deadlocks at compile time. - It works by associating a shared member variable with a synchronization primitive that protects it. The compiler can then check at each access if proper locking happened before. A good introduction are [0] and [1]. - TSA requires some help by the programmer via annotations. Luckily, LLVM's libcxx already has annotations for std::mutex, std::lock_guard, std::shared_mutex and std::scoped_lock. This commit enables them (--> contrib/libcxx-cmake/CMakeLists.txt). - Further, this commit adds convenience macros for the low-level annotations for use in ClickHouse (--> base/defines.h). For demonstration, they are leveraged in a few places. - As we compile with "-Wall -Wextra -Weverything", the required compiler flag "-Wthread-safety-analysis" was already enabled. Negative checks are an experimental feature of TSA and disabled (--> cmake/warnings.cmake). Compile times did not increase noticeably. - TSA is used in a few places with simple locking. I tried TSA also where locking is more complex. The problem was usually that it is unclear which data is protected by which lock :-(. But there was definitely some weird code where locking looked broken. So there is some potential to find bugs. *** Limitations of TSA besides the ones listed in [1]: - The programmer needs to know which lock protects which piece of shared data. This is not always easy for large classes. - Two synchronization primitives used in ClickHouse are not annotated in libcxx: (1) std::unique_lock: A releaseable lock handle often together with std::condition_variable, e.g. in solve producer-consumer problems. (2) std::recursive_mutex: A re-entrant mutex variant. Its usage can be considered a design flaw + typically it is slower than a standard mutex. In this commit, one std::recursive_mutex was converted to std::mutex and annotated with TSA. - For free-standing functions (e.g. helper functions) which are passed shared data members, it can be tricky to specify the associated lock. This is because the annotations use the normal C++ rules for symbol resolution. [0] https://clang.llvm.org/docs/ThreadSafetyAnalysis.html [1] https://static.googleusercontent.com/media/research.google.com/en//pubs/archive/42958.pdf
40 lines
1.1 KiB
C++
40 lines
1.1 KiB
C++
#pragma once
|
|
|
|
#include "SharedLibraryHandler.h"
|
|
#include <base/defines.h>
|
|
|
|
#include <unordered_map>
|
|
#include <mutex>
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
/// Each library dictionary source has unique UUID. When clone() method is called, a new UUID is generated.
|
|
/// There is a unique mapping from diciotnary UUID to sharedLibraryHandler.
|
|
class SharedLibraryHandlerFactory final : private boost::noncopyable
|
|
{
|
|
public:
|
|
static SharedLibraryHandlerFactory & instance();
|
|
|
|
SharedLibraryHandlerPtr get(const std::string & dictionary_id);
|
|
|
|
void create(
|
|
const std::string & dictionary_id,
|
|
const std::string & library_path,
|
|
const std::vector<std::string> & library_settings,
|
|
const Block & sample_block,
|
|
const std::vector<std::string> & attributes_names);
|
|
|
|
bool clone(const std::string & from_dictionary_id, const std::string & to_dictionary_id);
|
|
|
|
bool remove(const std::string & dictionary_id);
|
|
|
|
private:
|
|
/// map: dict_id -> sharedLibraryHandler
|
|
std::unordered_map<std::string, SharedLibraryHandlerPtr> library_handlers TSA_GUARDED_BY(mutex);
|
|
std::mutex mutex;
|
|
};
|
|
|
|
}
|