mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-10-09 18:10:48 +00:00
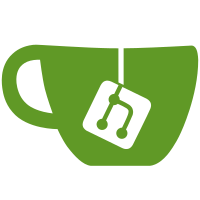
This is the first step of allowing heterogeneous cells in hash tables. performance test results are ``` 1. HashMap<UInt16, UInt8, TrivialHash, HashTableFixedGrower<16>>; 2. NewLookupMap<UInt16, UInt8> ResolutionWidth 30000 1 .................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................223550276.46 ResolutionWidth 30000 2 .................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................248772721.24 Best: 2 - 24877272124 ResolutionWidth 100000 1 ..........................................................................................................................................................................................................................................................238498413.99 ResolutionWidth 100000 2 ..........................................................................................................................................................................................................................................................261808889.98 Best: 2 - 26180888998 ResolutionWidth 300000 1 ...................................................................................239307348.81 ResolutionWidth 300000 2 ...................................................................................257592761.30 Best: 2 - 25759276130 ResolutionWidth 1000000 1 .........................240144759.26 ResolutionWidth 1000000 2 .........................257093531.91 Best: 2 - 25709353191 ResolutionWidth 5000000 1 .....241573260.35 ResolutionWidth 5000000 2 .....259314162.79 Best: 2 - 25931416279 ResolutionDepth 30000 1 .................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................217108119.84 ResolutionDepth 30000 2 .................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................................249459504.41 Best: 2 - 24945950441 ResolutionDepth 100000 1 ..........................................................................................................................................................................................................................................................229065162.17 ResolutionDepth 100000 2 ..........................................................................................................................................................................................................................................................253769105.64 Best: 2 - 25376910564 ResolutionDepth 300000 1 ...................................................................................233079225.18 ResolutionDepth 300000 2 ...................................................................................256316273.78 Best: 2 - 25631627378 ResolutionDepth 1000000 1 .........................234184633.51 ResolutionDepth 1000000 2 .........................261100491.57 Best: 2 - 26110049157 ResolutionDepth 5000000 1 .....233118795.66 ResolutionDepth 5000000 2 .....252436160.41 Best: 2 - 25243616041 ```
46 lines
1.2 KiB
C++
46 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include <Common/HashTable/ClearableHashSet.h>
|
|
#include <Common/HashTable/FixedHashTable.h>
|
|
|
|
|
|
template <typename Key>
|
|
struct FixedClearableHashTableCell
|
|
{
|
|
using State = ClearableHashSetState;
|
|
|
|
using value_type = Key;
|
|
UInt32 version;
|
|
|
|
FixedClearableHashTableCell() {}
|
|
FixedClearableHashTableCell(const Key &, const State & state) : version(state.version) {}
|
|
|
|
bool isZero(const State & state) const { return version != state.version; }
|
|
void setZero() { version = 0; }
|
|
static constexpr bool need_zero_value_storage = false;
|
|
void setMapped(const value_type & /*value*/) {}
|
|
|
|
struct CellExt
|
|
{
|
|
Key key;
|
|
value_type & getValueMutable() { return key; }
|
|
const value_type & getValue() const { return key; }
|
|
void update(Key && key_, FixedClearableHashTableCell *) { key = key_; }
|
|
};
|
|
};
|
|
|
|
|
|
template <typename Key, typename Allocator = HashTableAllocator>
|
|
class FixedClearableHashSet : public FixedHashTable<Key, FixedClearableHashTableCell<Key>, Allocator>
|
|
{
|
|
public:
|
|
using key_type = Key;
|
|
using value_type = typename FixedClearableHashSet::cell_type::value_type;
|
|
|
|
void clear()
|
|
{
|
|
++this->version;
|
|
this->m_size = 0;
|
|
}
|
|
};
|