mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-20 08:40:50 +00:00
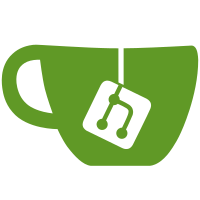
* Added a new type of Exception for better recognition of connection failures * Added more logging for mysql communication * Retries on connection loss during query. Make MySQL dictionary source resilient to unexpected loss of connection during querying MySQL. This behavior is controlled with ".fail_on_connection_loss" config parameter, which defaults to false. * Stripped some debugging leftover garbage * Minor followup corrections * Corrections after PR comments * Yet more fixes
63 lines
2.0 KiB
C++
63 lines
2.0 KiB
C++
#pragma once
|
|
|
|
#include <sstream>
|
|
#include <Poco/Exception.h>
|
|
#include <mysqlxx/Types.h>
|
|
|
|
|
|
namespace mysqlxx
|
|
{
|
|
/// Common exception class for MySQL library. Functions code() and errnum() return error numbers from MySQL, for details see mysqld_error.h
|
|
struct Exception : public Poco::Exception
|
|
{
|
|
Exception(const std::string & msg, int code = 0) : Poco::Exception(msg, code) {}
|
|
int errnum() const { return code(); }
|
|
const char * name() const throw() override { return "mysqlxx::Exception"; }
|
|
const char * className() const throw() override { return "mysqlxx::Exception"; }
|
|
};
|
|
|
|
|
|
/// Cannot connect to MySQL server
|
|
struct ConnectionFailed : public Exception
|
|
{
|
|
ConnectionFailed(const std::string & msg, int code = 0) : Exception(msg, code) {}
|
|
const char * name() const throw() override { return "mysqlxx::ConnectionFailed"; }
|
|
const char * className() const throw() override { return "mysqlxx::ConnectionFailed"; }
|
|
};
|
|
|
|
|
|
/// Connection to MySQL server was lost
|
|
struct ConnectionLost : public Exception
|
|
{
|
|
ConnectionLost(const std::string & msg, int code = 0) : Exception(msg, code) {}
|
|
const char * name() const throw() override { return "mysqlxx::ConnectionLost"; }
|
|
const char * className() const throw() override { return "mysqlxx::ConnectionLost"; }
|
|
};
|
|
|
|
|
|
/// Erroneous query.
|
|
struct BadQuery : public Exception
|
|
{
|
|
BadQuery(const std::string & msg, int code = 0) : Exception(msg, code) {}
|
|
const char * name() const throw() override { return "mysqlxx::BadQuery"; }
|
|
const char * className() const throw() override { return "mysqlxx::BadQuery"; }
|
|
};
|
|
|
|
|
|
/// Value parsing failure
|
|
struct CannotParseValue : public Exception
|
|
{
|
|
CannotParseValue(const std::string & msg, int code = 0) : Exception(msg, code) {}
|
|
const char * name() const throw() override { return "mysqlxx::CannotParseValue"; }
|
|
const char * className() const throw() override { return "mysqlxx::CannotParseValue"; }
|
|
};
|
|
|
|
|
|
std::string errorMessage(MYSQL * driver);
|
|
|
|
/// For internal need of library.
|
|
void checkError(MYSQL * driver);
|
|
[[noreturn]] void onError(MYSQL * driver);
|
|
|
|
}
|