mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-09-29 05:00:47 +00:00
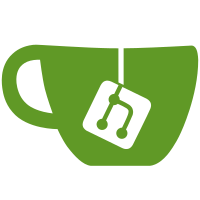
After first INSERT for disk2 there should not be created any per-shard directory, so find(1) should report an error, like: find: '/disk2/data/test/dist_foo/default@127%2E0%2E0%2E2:9000': No such file or directory" But sometimes output can be fixed, and output of wc(1) will goes first and python's int() will parse it and not fail, but if find(1) stderr will goes first the int() will fail to parse. And here is an example of such mixing: $ docker run --name alpine --rm -it alpine top $ docker exec alpine sh -c 'echo foo >&2 | wc -c' foo 0 $ docker exec alpine sh -c 'echo foo >&2 | wc -c' 0 foo
67 lines
2.1 KiB
Python
67 lines
2.1 KiB
Python
# pylint: disable=unused-argument
|
|
# pylint: disable=redefined-outer-name
|
|
# pylint: disable=line-too-long
|
|
|
|
import pytest
|
|
|
|
from helpers.cluster import ClickHouseCluster
|
|
|
|
cluster = ClickHouseCluster(__file__)
|
|
|
|
node = cluster.add_instance('node',
|
|
config_dir='configs',
|
|
tmpfs=['/disk1:size=100M', '/disk2:size=100M'])
|
|
|
|
@pytest.fixture(scope='module')
|
|
def start_cluster():
|
|
try:
|
|
cluster.start()
|
|
node.query('CREATE DATABASE test ENGINE=Ordinary')
|
|
yield cluster
|
|
finally:
|
|
cluster.shutdown()
|
|
|
|
def _files_in_dist_mon(node, root, table):
|
|
return int(node.exec_in_container([
|
|
'bash',
|
|
'-c',
|
|
# `-maxdepth 1` to avoid /tmp/ subdirectory
|
|
'find /{root}/data/test/{table}/default@127%2E0%2E0%2E2:9000 -maxdepth 1 -type f 2>/dev/null | wc -l'.format(root=root, table=table)
|
|
]).split('\n')[0])
|
|
|
|
def test_different_versions(start_cluster):
|
|
node.query('CREATE TABLE test.foo (key Int) Engine=Memory()')
|
|
node.query("""
|
|
CREATE TABLE test.dist_foo (key Int)
|
|
Engine=Distributed(
|
|
test_cluster_two_shards,
|
|
test,
|
|
foo,
|
|
key%2,
|
|
'default'
|
|
)
|
|
""")
|
|
# manual only (but only for remote node)
|
|
node.query('SYSTEM STOP DISTRIBUTED SENDS test.dist_foo')
|
|
|
|
node.query('INSERT INTO test.dist_foo SELECT * FROM numbers(100)')
|
|
assert _files_in_dist_mon(node, 'disk1', 'dist_foo') == 1
|
|
assert _files_in_dist_mon(node, 'disk2', 'dist_foo') == 0
|
|
|
|
assert node.query('SELECT count() FROM test.dist_foo') == '100\n'
|
|
node.query('SYSTEM FLUSH DISTRIBUTED test.dist_foo')
|
|
assert node.query('SELECT count() FROM test.dist_foo') == '200\n'
|
|
|
|
#
|
|
# RENAME
|
|
#
|
|
node.query('RENAME TABLE test.dist_foo TO test.dist2_foo')
|
|
|
|
node.query('INSERT INTO test.dist2_foo SELECT * FROM numbers(100)')
|
|
assert _files_in_dist_mon(node, 'disk1', 'dist2_foo') == 0
|
|
assert _files_in_dist_mon(node, 'disk2', 'dist2_foo') == 1
|
|
|
|
assert node.query('SELECT count() FROM test.dist2_foo') == '300\n'
|
|
node.query('SYSTEM FLUSH DISTRIBUTED test.dist2_foo')
|
|
assert node.query('SELECT count() FROM test.dist2_foo') == '400\n'
|