mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-10-01 06:00:49 +00:00
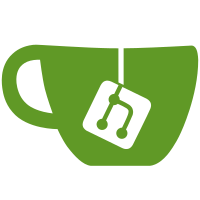
* ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * Fixed error in ColumnArray::replicateGeneric [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150]. * ColumnConst: unification (incomplete) [#CLICKHOUSE-3150].
88 lines
1.8 KiB
C++
88 lines
1.8 KiB
C++
#pragma once
|
|
|
|
#include <Common/typeid_cast.h>
|
|
#include <DataTypes/IDataType.h>
|
|
#include <Columns/IColumn.h>
|
|
#include <Columns/ColumnConst.h>
|
|
|
|
|
|
|
|
namespace DB
|
|
{
|
|
|
|
/// Methods, that helps dispatching over real column types.
|
|
|
|
template <typename Type>
|
|
const Type * checkAndGetDataType(const IDataType * data_type)
|
|
{
|
|
return typeid_cast<const Type *>(data_type);
|
|
}
|
|
|
|
template <typename Type>
|
|
bool checkDataType(const IDataType * data_type)
|
|
{
|
|
return checkAndGetDataType<Type>(data_type);
|
|
}
|
|
|
|
|
|
template <typename Type>
|
|
const Type * checkAndGetColumn(const IColumn * column)
|
|
{
|
|
return typeid_cast<const Type *>(column);
|
|
}
|
|
|
|
template <typename Type>
|
|
bool checkColumn(const IColumn * column)
|
|
{
|
|
return checkAndGetColumn<Type>(column);
|
|
}
|
|
|
|
|
|
template <typename Type>
|
|
const ColumnConst * checkAndGetColumnConst(const IColumn * column)
|
|
{
|
|
if (!column->isConst())
|
|
return {};
|
|
|
|
const ColumnConst * res = static_cast<const ColumnConst *>(column);
|
|
|
|
if (!checkColumn<Type>(&res->getDataColumn()))
|
|
return {};
|
|
|
|
return res;
|
|
}
|
|
|
|
template <typename Type>
|
|
const Type * checkAndGetColumnConstData(const IColumn * column)
|
|
{
|
|
const ColumnConst * res = checkAndGetColumnConst<Type>(column);
|
|
|
|
if (!res)
|
|
return res;
|
|
|
|
return &res->getDataColumn();
|
|
}
|
|
|
|
template <typename Type>
|
|
const bool checkColumnConst(const IColumn * column)
|
|
{
|
|
return checkAndGetColumnConst<Type>(column);
|
|
}
|
|
|
|
|
|
/// Returns non-nullptr if column is ColumnConst with ColumnString or ColumnFixedString inside.
|
|
const ColumnConst * checkAndGetColumnConstStringOrFixedString(const IColumn * column);
|
|
|
|
|
|
/// Transform anything to Field.
|
|
template <typename T>
|
|
inline Field toField(const T & x)
|
|
{
|
|
return Field(typename NearestFieldType<T>::Type(x));
|
|
}
|
|
|
|
|
|
ColumnPtr convertConstTupleToTupleOfConstants(const ColumnConst & column);
|
|
|
|
}
|