mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-10-01 06:00:49 +00:00
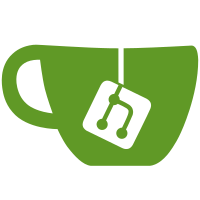
* Every function in its own file, part 7 [#CLICKHOUSE-2] * Every function in its own file, part 7 [#CLICKHOUSE-2] * Every function in its own file, part 7 [#CLICKHOUSE-2] * Every function in its own file, part 7 [#CLICKHOUSE-2] * Every function in its own file, part 7 [#CLICKHOUSE-2] * Fixed build #3666
62 lines
1.9 KiB
C++
62 lines
1.9 KiB
C++
#include <Functions/FunctionFactory.h>
|
|
#include <Functions/FunctionBinaryArithmetic.h>
|
|
#include <Core/AccurateComparison.h>
|
|
|
|
namespace DB
|
|
{
|
|
|
|
template <typename A, typename B>
|
|
struct LeastBaseImpl
|
|
{
|
|
using ResultType = NumberTraits::ResultOfLeast<A, B>;
|
|
|
|
template <typename Result = ResultType>
|
|
static inline Result apply(A a, B b)
|
|
{
|
|
/** gcc 4.9.2 successfully vectorizes a loop from this function. */
|
|
return static_cast<Result>(a) < static_cast<Result>(b) ? static_cast<Result>(a) : static_cast<Result>(b);
|
|
}
|
|
|
|
#if USE_EMBEDDED_COMPILER
|
|
static constexpr bool compilable = true;
|
|
|
|
static inline llvm::Value * compile(llvm::IRBuilder<> & b, llvm::Value * left, llvm::Value * right, bool is_signed)
|
|
{
|
|
if (!left->getType()->isIntegerTy())
|
|
/// XXX minnum is basically fmin(), it may or may not match whatever apply() does
|
|
return b.CreateMinNum(left, right);
|
|
return b.CreateSelect(is_signed ? b.CreateICmpSLT(left, right) : b.CreateICmpULT(left, right), left, right);
|
|
}
|
|
#endif
|
|
};
|
|
|
|
template <typename A, typename B>
|
|
struct LeastSpecialImpl
|
|
{
|
|
using ResultType = std::make_signed_t<A>;
|
|
|
|
template <typename Result = ResultType>
|
|
static inline Result apply(A a, B b)
|
|
{
|
|
static_assert(std::is_same_v<Result, ResultType>, "ResultType != Result");
|
|
return accurate::lessOp(a, b) ? static_cast<Result>(a) : static_cast<Result>(b);
|
|
}
|
|
|
|
#if USE_EMBEDDED_COMPILER
|
|
static constexpr bool compilable = false; /// ???
|
|
#endif
|
|
};
|
|
|
|
template <typename A, typename B>
|
|
using LeastImpl = std::conditional_t<!NumberTraits::LeastGreatestSpecialCase<A, B>, LeastBaseImpl<A, B>, LeastSpecialImpl<A, B>>;
|
|
|
|
struct NameLeast { static constexpr auto name = "least"; };
|
|
using FunctionLeast = FunctionBinaryArithmetic<LeastImpl, NameLeast>;
|
|
|
|
void registerFunctionLeast(FunctionFactory & factory)
|
|
{
|
|
factory.registerFunction<FunctionLeast>();
|
|
}
|
|
|
|
}
|