mirror of
https://github.com/ClickHouse/ClickHouse.git
synced 2024-12-15 10:52:30 +00:00
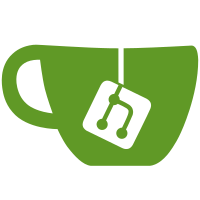
Since HashMap creates 2^8 elements by default, while dense_hash_map should be good here. Signed-off-by: Azat Khuzhin <a.khuzhin@semrush.com>
51 lines
1.1 KiB
C++
51 lines
1.1 KiB
C++
#include <Interpreters/Context.h>
|
|
#include <Common/tests/gtest_global_context.h>
|
|
#include <gtest/gtest.h>
|
|
#include <thread>
|
|
|
|
using namespace DB;
|
|
|
|
template <typename Ptr>
|
|
void run(Ptr context)
|
|
{
|
|
for (size_t i = 0; i < 100; ++i)
|
|
{
|
|
std::thread t1([context]
|
|
{
|
|
if constexpr (std::is_same_v<ContextWeakPtr, Ptr>)
|
|
context.lock()->getAsyncReadCounters();
|
|
else
|
|
context->getAsyncReadCounters();
|
|
});
|
|
|
|
std::thread t2([context]
|
|
{
|
|
Context::createCopy(context);
|
|
});
|
|
|
|
t1.join();
|
|
t2.join();
|
|
}
|
|
}
|
|
|
|
TEST(Context, MutableRace)
|
|
{
|
|
auto context = Context::createCopy(getContext().context);
|
|
context->makeQueryContext();
|
|
run<ContextMutablePtr>(context);
|
|
}
|
|
|
|
TEST(Context, ConstRace)
|
|
{
|
|
auto context = Context::createCopy(getContext().context);
|
|
context->makeQueryContext();
|
|
run<ContextPtr>(context);
|
|
}
|
|
|
|
TEST(Context, WeakRace)
|
|
{
|
|
auto context = Context::createCopy(getContext().context);
|
|
context->makeQueryContext();
|
|
run<ContextWeakPtr>(context);
|
|
}
|